Sergey Leschev
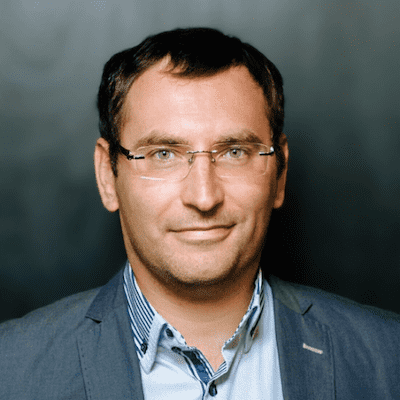
FullStack Developer
Swift (L6+) | Full Stack (L6+) | Design Patterns (L6+) | System Architect (L7+) | Blog
Google Engineering Level: L6+
đ Awards
Ranking #Dev: Global TOP 200 (Certificate)
Languages: TypeScript, Shell, Database (T-SQL, PL/SQL, MySQL), Concurrency (Python3).
Algorithms: linked lists, binary search, hash table, queue/stack, dfs/bfs, sort, heap/hash, two pointers, sliding window, tree, greedy problems etc.
Golden Award for the Year of the Tiger Challenge

Algorithmic skills: Dynamic programming, Greedy algorithms, Catepillar method, Binary search algorithm, Fibonacci numbers, Euclidean algorithm, Sieve of Eratosthenes, Prime and composite numbers, Maximum slice problem, Stack and Queues, Sorting, Time Complexity, Arrays, Prefix Sums, Leader, etc.
Contest: Algorithms, SQL, Data Structures, Bitwise operations (bit-ops), Frontend.
Tapping on a link will take you to relevant certificates.
Front-End
- [X] Basics
- [X] HTML
- [X] Learn the basics of HTML
- [X] Make a few pages as an exercise
- [X] CSS
- [X] Learn the basics of CSS
- [X] Style pages from previous step
- [X] Build a page with grid and flexbox
- [X] JS Basics
- [X] Get familiar with the syntax
- [X] Learn basic operations on DOM
- [X] Learn mechanisms typical for JS (Hoisting, Event Bubbling, Prototyping)
- [X] Make some AJAX (XHR) calls
- [X] Learn new features (ECMA Script 6+)
- [X] Additionally, get familiar with the jQuery library
- [X] HTML
- [X] General Development Skills
- [X] Learn GIT, create a few repositories on GitHub, share your code with other people
- [X] Know HTTP(S) protocol, request methods (GET, POST, PUT, PATCH, DELETE, OPTIONS)
- [X] Get familiar with terminal, configure your shell (bash, zsh, fish)
- [X] Studied algorithms and data structures in the university
- [X] Studied design patterns in the university
- [X] Learn React on official website or complete some courses
- [X] Get familiar with tools that you will be using
- [X] Styling
- [X] CSS Preprocessor
- [X] CSS Frameworks
- [X] Bootstrap
- [X] Materialize, Material UI, Material Design Lite
- [X] Bulma
- [X] Semantic UI
- [X] CSS Architecture
- [X] CSS in JS
- [X] Styled Components
- [X] Radium
- [X] Emotion
- [X] JSS
- [X] Aphrodite
- [X] State Management
- [X] Component State/Context API
- [X] Redux
- [X] Async actions (Side Effects)
- [X] Redux Thunk
- [X] Redux Better Promise
- [X] Redux Saga
- [X] Redux Observable
- [X] Helpers
- [X] Data persistence
- [X] Redux Persist
- [X] Redux Phoenix
- [X] Redux Form
- [X] Async actions (Side Effects)
- [X] Type Checkers
- [X] PropTypes
- [X] TypeScript
- [X] Flow
- [X] Form Helpers
- [X] Redux Form
- [X] Formik
- [X] Formsy
- [X] Final Form
- [X] Routing
- [X] React-Router
- [X] Router5
- [X] Redux-First Router
- [X] Reach Router
- [X] API Clients
- [X] Utility Libraries
- [X] Lodash
- [X] Moment
- [X] classnames
- [X] Numeral
- [X] RxJS
- [X] ImmutableJS
- [X] Ramda
- [X] Testing
- [X] Internationalization
- [X] React Intl
- [X] React i18next
- [X] Server Side Rendering
- [X] Static Site Generator
- [X] Gatsby
- [X] Backend Framework Integration
- [X] React on Rails
- [X] Mobile
- [X] React Native
- [X] Desktop
- [X] Electron
- [X] React Native Windows
Back-End
-
[X] Prerequisites
- [X] JavaScript
- [X] NPM
- [X] Node.js
- [X] ECMAScript
-
[X] General Development Skills
- [X] Learn GIT, create a few repositories on GitHub, share your code with other people
- [X] Know HTTP(S) protocol, request methods (GET, POST, PUT, PATCH, DELETE, OPTIONS)
- [X] Studied algorithms and data structures in the university
- [X] Studied design patterns in the university
- [X] Clean code
-
[X] Web Frameworks
- [X] Express.js
- [X] Adonis.js
- [X] Meteor.js
- [X] Nest.js
- [X] Sails.js
- [X] Koa.js
- [X] Loopback.io
- [X] egg.js
- [X] midway
-
[X] Databases
- [X] Relational
- [X] SQL Server
sources.sql
- [X] PostgreSQL
sources.sql
- [X] MariaDB
sources.sql
- [X] MySQL
sources.sql
- [X] SQL Server
- [X] Cloud Databases
- [X] Search Engines
- [X] ElasticSearch
- [X] Solr
- [X] Sphinx
- [X] NoSQL
- [X] Relational
-
[X] Caching
- [X] Node-Cache
- [X] Distributed Cache
- [X] Memory Cache
-
[X] Logging
- [X] Log Frameworks
- [X] Node-Loggly
- [X] Winston
- [X] Node-Bunyan
- [X] Morgan
- [X] Log Management System
- [X] Sentry.io
- [X] Loggly.com
- [X] Log Frameworks
-
[X] Template Engines
- [X] Mustache.js
- [X] Handlebars
- [X] EJS
- [X] Pug
- [X] Nunjunks.js
-
[X] Real-Time Communication
- [X] Socket.IO
-
[X] Typed Superset
- [X] TypeScript
- [X] Flow
-
[X] API Clients
- [X] REST
- [X] Node-Rest-Client
- [X] Axios
- [X] GraphQL
- [X] Authentication
- [X] REST
-
[X] Good to Know
- [X] Async.js
- [X] PM2
- [X] Commander.js
- [X] Passport
- [X] Nodemailer
- [X] Marked
- [X] ESLint
-
[X] Testing
-
[X] Task Scheduling
- [X] Agenda
- [X] Cronicle
- [X] Node-Schedule
-
[X] MicroServices
- [X] Message-Broker
- [X] RabbitMQ
- [X] Apache Kafka
- [X] ActiveMQ
- [X] Azure Service Bus
- [X] Message-Bus
- [X] Message-Broker
-
[X] Design-Patterns
Computer Science
- [X]
Computer Science knowledge
- [X]
Algorithms
cert
- [X]
Sorting
cert
- [X]
Graph Theory
cert
- [X]
Strings
cert
- [X] Greedy
cert
- [X] Dynamic Programming
cert
- [X] Bit Manipulation
cert
- [X]
Recursion
cert
- [X] Game Theory
cert
- [X] NP Complete
cert
- [X]
Big-O notation
cert
- [X]
- [X]
Abstract Data Types
cert
- [X]
System design
- [X]
React Custom Hooks (L6+)
useArray useAsync useClickOutside useCookie useCopyToClipboard useDarkMode useDebounce useDebugInformation useDeepCompareEffect useEffectOnce useEventListener useFetch useGeolocation useHover useLongPress useMediaQuery useOnlineStatus useOnScreen usePrevious useRenderCount useScript useStateWithHistory useStateWithValidation useStorage useTimeout useToggle useTranslation useUpdateEffect useWindowSize
Design Patterns (L6+)
Project Guidelines (L6+)
A set of best practices in my projects.
- [X] Git
- [X] Documentation
- [X] Environments
- [X] Dependencies
- [X] Tesing
- [X] Structure and Naming
- [X] Code Style
- [X] Logging
- [X] API
Licenses & certifications
- đ LeetCode Global TOP 200 (TypeScript: Certificate, Sources: TypeScript).
- đ Golden Award for the Year of the Tiger Challenge (TypeScript: Certificate, Sources: Codility).
- 2022 July LeetCode Challenge (2022-07-31).
- 2022 June LeetCode Challenge (2022-06-30).
- 2022 May LeetCode Challenge (2022-05-31).
- 2022 Apr LeetCode Challenge (2022-04-30).
- LeetCode Dynamic Programming (2022-05-07).
- Graph Theory (2022-04-30).
- SQL (2022-04-26).
- Algorithm I (2022-04-30), Algorithm II (2022-05-21).
- Data Structure I (2022-04-30), Data Structure II (2022-05-21).
- Binary Search I (2022-04-28), Binary Search II (2022-05-18).
- Programming Skills I (2022-04-28), Programming Skills II (2022-05-18).
- LinkedIn Skill Asessment (Front-End): Front-end Development, Angular, React, Javascript, HTML, CSS, jQuery.
- LinkedIn Skill Asessment (Back-End): Node.js, Java, Spring Framework, Scala, C#, .NET Framework, Unity, Python (Programming Language), Django, PHP, C (Programming Language).
- LinkedIn Skill Asessment (Databases): MongoDB, NoSQL, Transact-SQL (T-SQL), MySQL.
- LinkedIn Skill Asessment (Infra/DevOps): Bash, Git, Amazon Web Services (AWS), AWS Lambda, Google Cloud Platform (GCP), Microsoft Azure, Hadoop, IT Operations.
Latest Projects
[Web] Health & Fitness Web App
Role: Team Lead (L6+).
Description (tasks): Development architecture and new features.
Tech Stack:
- React 18+: Hooks. Redux. Redux Toolkit.
- UI Framework: PrimeReact.
- NodeJS: NestJS, TypeORM.
- PostgreSQL. ORM.
- MongoDB. Aggregations.
- Docker.
- CI/CD (DO, AWS, Github Actions).
- gRPC, WebSockets.
Contacts
I have a clear focus on time-to-market and don't prioritize technical debt. And I took part in the Pre-Sale/RFX activity as a System Architect, assessment efforts for Frontend (React-TypeScript) and Backend (NodeJS-.NET-PHP-Kafka-SQL-NoSQL). And I also formed the work of Pre-Sale as a CTO from Opportunity to Proposal via knowledge transfer to Successful Delivery.
đŠī¸ #startups #management #cto #swift #typescript #database
đ§ Email: sergey.leschev@gmail.com
đ LinkedIn: https://linkedin.com/in/sergeyleschev
đ Twitter: https://twitter.com/sergeyleschev
đ Github: https://github.com/sergeyleschev
đ Website: https://sergeyleschev.github.io
đ DEV Community: https://dev.to/sergeyleschev
đ Reddit: https://reddit.com/user/sergeyleschev
đ Quora: https://quora.com/sergey-leschev
đ Medium: https://medium.com/@sergeyleschev
đ¨ī¸ PDF: Download
ALT: SIARHEI LIASHCHOU