Sergey Leschev
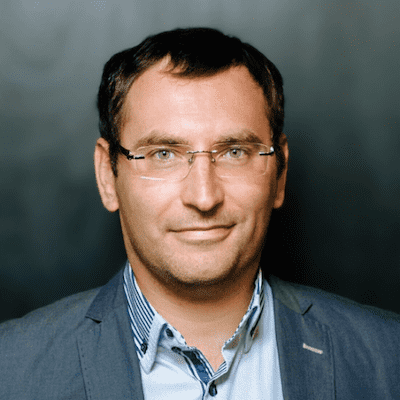
iOS Developer
Swift (L6+) | Full Stack (L6+) | Design Patterns (L6+) | System Architect (L7+) | Blog
Google Engineering Level: L6+
π Awards
Ranking #Dev: Global TOP 200 (Certificate)
Languages: Swift, Shell, Database (T-SQL, PL/SQL, MySQL), Concurrency (Python3).
Algorithms: linked lists, binary search, hash table, queue/stack, dfs/bfs, sort, heap/hash, two pointers, sliding window, tree, greedy problems etc.
Golden Award MuadβDibβs Challenge
Languages: Swift.
Algorithmic skills: Dynamic programming, Greedy algorithms, Binary search, Stack and Queues, Sorting, Time Complexity.
Contest: Algorithms, Data Structures.
Tapping on a link will take you to relevant certificates.
iOS
- [X]
Languages
- [X]
Swift
- [X] Closures
- [X]
Initializers
- [X] Generics
- [X]
Protocols
- [X]
Structs
- [X]
Enums
- [X] Runtime
- [X] Method dispatch
- [X]
- [X]
Memory management
- [X]
Stack and Heap
- [X]
Value vs Reference type
- [X] MRC
- [X]
ARC
- [X]
- [X] Weak references
- [X]
Retain cycles
- [X] Garbage collection
- [X]
Memory leaks
- [X] Shallow and deep copying
- [X] Autorelease pool
- [X]
- [X]
Multithreading and concurrency
- [X] POSIX and NSThreads
- [X] Perform selector family
- [X]
GCD
- [X]
NSOperation(Queue)
- [X]
Runloop
- [X]
Synchronization
- [X] Problems
- [X] Race condition
- [X] Deadlock
- [X] Readersβwriters problem
- [X]
Cocoa Touch
- [X]
UIKit
- [X]
UIApplication
- [X]
States
- [X] UIApplicationDelegate
- [X]
- [X]
UIViews
- [X]
UITableViews
- [X]
UICollectionViews
- [X]
- [X] Layers
- [X]
Layout
- [X]
Frame-based
- [X]
Autolayout
- [X] UIStackView
- [X]
- [X] Animations
- [X] Transform
- [X]
Navigation
- [X]
UIViewController
- [X]
Lifecycle
- [X]
- [X]
- [X]
Foundation
- [X]
Notifications vs Delegation vs Observing
- [X] Collections
- [X]
Networking
- [X]
Serialization
- [X]
- [X] UserNotifications
- [X] Core Location
- [X] Core Motion
- [X]
Work in background mode
- [X]
- [X]
Software Architecture
- [X]
Design Patterns
- [X]
Cocoa
- [X] Abstract Factory
- [X] Class cluster
- [X] Adapter
- [X] Command Pattern
- [X] Chain of Responsibility
- [X] Decorator
- [X] Delegation
- [X] Categories
- [X] Facade
- [X] Memento
- [X] Observer
- [X] Proxy
- [X] Receptionist
- [X] Singleton
- [X] Template Method
- [X] MVC
- [X] Abstract Factory
- [X]
Architectural
- [X]
MVC
- [X]
MVVM
/UIKitPlus
- [X]
MVP
- [X]
Clean architecture
- [X] Coordinators
- [X]
- [X]
Creational
- [X] Factory
- [X] Abstract Factory
- [X] Builder
- [X] Factory Method
- [X] Object Pool
- [X] Prototype
- [X] Singleton
- [X]
Structural
- [X] Adapter
- [X] Bridge
- [X] Composite
- [X] Decorator
- [X] Facade
- [X] Flyweight
- [X] Proxy
- [X]
Behavioural
- [X] Command
- [X] Chain of responsibility
- [X] Interpreter
- [X] Iterator
- [X] Mediator
- [X] Memento
- [X] Observer
- [X] State
- [X] Strategy
- [X] Visitor
- [X] Concurrency
- [X] Anti-pattern
- [X]
- [X]
Design Principles
- [X]
SOLID
- [X]
Inversion of Control
- [X]
Dependency Injection
- [X] Service Locator
- [X]
- [X] Protocol-Oriented Programming
- [X]
- [X]
- [X]
Dependencies management
- [X] Cocoapods
- [X] Carthage
- [X] Swift Package Manager
- [X] Project structure and File/Group organisation
- [X]
Version Control Systems
- [X]
Git
- [X]
- [X] Debugging
- [X] Instruments
- [X] Best practices
- [X] Checklists
- [X] UX
- [X]
Caching and Persistency
- [X]
Testing
- [X]
Unit Tests
- [X] Snapshot Tests
- [X] Functional test
- [X] UI Tests
- [X] TDD
- [X] BDD
- [X]
- [X] Performance optimization
- [X] Increase FPS
- [X] Decrease memory footprint
- [X] Code signing
- [X] Tools
- [X] IDE
- [X] Xcode
- [X] Swiftlint
- [X] Sourcery
- [X] Fastlane
- [X] Charles
- [X] IDE
- [X] Continuous Integration
- [X] Github Actions / Jenkins
- [X] Xcode server
- [X] Security
- [X] Keychain
- [X] tvOS
- [X] WatchKit
- [X] Programming Paradigms
- [X] Object-Oriented
- [X] Functional
- [X] Functional Reactive Programming Frameworks
- [X] React Native
- [X] RxSwift
- [X] RxRealm, RxDataSources
- [X] Combine
- [X] Functional Reactive Programming Frameworks
Computer Science
- [X]
Computer Science knowledge
- [X]
Algorithms
cert
- [X]
Sorting
cert
- [X]
Graph Theory
cert
- [X]
Strings
cert
- [X] Greedy
cert
- [X] Dynamic Programming
cert
- [X] Bit Manipulation
cert
- [X]
Recursion
cert
- [X] Game Theory
cert
- [X] NP Complete
cert
- [X]
Big-O notation
cert
- [X]
- [X]
Abstract Data Types
cert
- [X]
System design
- [X]
Design Patterns (L6+)
Frameworks
Alamofire, Combine, CoreAnimation, GoogleMaps, Moya, Pushy, ReactiveSwift, RxSwift, SnapKit, StoreKit, Π‘oordinators, SwiftUI, SwiftLint, SwiftCentrifuge, Swift Package Manager, AppsFlyer, ApplePay, AdSupport, Autolayout, CGD, Clean, Clean Code, CocoaPods, CoreData, CoreLocation, Crashlytics, DRY, Fabric, Firebase, Firebase Analytics, Firebase Remote Config, Foundation, graphql, gRPC, iOS Human Interface Guidelines, JSON, jwt, KISS, MessageUI, Monorepo, MVC, MVP, MVVM, NSOperation, oauth, OpenAPI, Swagger, Storyboard, sqlite, SOLID, Socket.IO, SOAP, RIBs, REST, rest, Realm, TestFlight, UI Testing, UIKit, unit, URLSession, Viper, WebKit, XCTest, XML.
Project Guidelines (L6+)
A set of best practices in my projects.
- [X] Git
- [X] Documentation
- [X] Environments
- [X] Code Style
- [X] Logging
- [X] API
Licenses & certifications
- π LeetCode Global TOP 200 (Swift: Certificate, Sources: Swift).
- π Golden Award MuadβDibβs Challenge (Swift: Certificate, Sources: Swift).
- 2022 July LeetCode Challenge (2022-07-31).
- 2022 June LeetCode Challenge (2022-06-30).
- 2022 May LeetCode Challenge (2022-05-31).
- 2022 Apr LeetCode Challenge (2022-04-30).
- LeetCode Dynamic Programming (2022-05-07).
- Graph Theory (2022-04-30).
- SQL (2022-04-26).
- Algorithm I (2022-04-30), Algorithm II (2022-05-21).
- Data Structure I (2022-04-30), Data Structure II (2022-05-21).
- Binary Search I (2022-04-28), Binary Search II (2022-05-18).
- Programming Skills I (2022-04-28), Programming Skills II (2022-05-18).
- LinkedIn Skill Asessment (Mobile): Swift (Programming Language), Object-Oriented Programming (OOP), Objective-C, C++, Ionic, JSON, XML, Android, Kotlin, Maven, Java, REST APIs.
- ο£Ώ Health & Fitness iOS App / Fitness Motivation / AppStore / Sources: SwiftUI @ S. Leschev.
- ο£Ώ Social Network iOS App / Social Network / AppStore / Sources: SwiftUI @ S. Leschev.
- ο£Ώ Utility MacOS App / Calc-It / Core / Sources: Swift @ S. Leschev.
Latest Projects
[ο£Ώ iOS] Live Streaming App in UIKit with VIPER.
Role: Senior iOS Developer, Team Lead (L6+).
Description (tasks): Development architecture and new features.
Tech Stack:
- Swift 5+.
- VIPER (Dependency Injection, Assembly, Services, Interactor, Presenter, State, Adapter) + MVVM (Combine, PromiseKit).
- Alamofire, Decodable, Combine.
- GCD/Operations.
- Agora Video SDK, Chat SDK, Beautification SDK. WebRTC. GRPC.
- Modular architecture (Frameworks, Development Pods).
- SwiftGen (Localization, Image, Colors).
- SwiftLint.
- Auth: Facebook, Google, Apple ID.
- Firebase, Crashlytics, Amplitude, AppsFlyer.
- Push-notifications (Firebase).
- UIKit, Autolayout, Core Animations, Skeleton, Lottie.
- Git (Flow, CodeReview), Fastlane, Figma.
[ο£Ώ iOS] Fitness iOS App in UIKit with Clean Swift.
Role: Senior iOS Developer, Team Lead (L6+).
Description (tasks): Development architecture and new features.
Tech Stack:
- Swift 5+.
- Clean Swift Architecture.
- Alamofire, ObjectMapper.
- GCD/Operations.
- AVFoundation, Streaming: HLS (Cloudflare/nginx).
- AirPlay [Composition (video+audio), Secondary Display].
- Realm.
- Modular architecture (Frameworks, Development Pods).
- SwiftGen (Localization, Image, Colors).
- SwiftLint.
- Auth: Facebook, Google, Apple ID, Fitbit.
- Amplitude, Crashlytics, AppsFlyer (+OneLink).
- Analytics: Facebook (SKAd + Conversions API).
- Push-notifications (OneSignal).
- UIKit, Autolayout, Core Animations, Lottie.
- Git (Flow, CodeReview), Zeplin, Figma, Sketch.
[ο£Ώ iOS] Social Network App in SwiftUI with MVVM.
Role: iOS Developer (L6+).
Description (tasks): Development architecture and new features.
Tech Stack:
- SwiftUI.
- Custom UI components.
- URLSession.
- Firebase for Login/Register.
- Push Notifications.
- GCD/Operations.
- Git, Figma, Sketch.
Sources: SwiftUI
[ο£Ώ iOS] Fitness Motivation Coach App in SwiftUI.
Role: iOS Developer (L6+).
Description (tasks): Development architecture and new features.
Tech Stack:
- SwiftUI.
- Watch Extension (WatchOS).
- AppClip Extension.
- Widget (iOS 14).
- ObjectMapper.
- URLSession.
- Keychain.
- Lottie.
- Push Notifications.
- GCD/Operations.
- Git, Figma, Sketch.
Website: motivations.coach
Sources: SwiftUI
Apple Developer Academy
Worldwide Developers Conference 2023 (WWDC23)
- Swift & SwiftUI.
- ActivityKit.
- Spotlight apps with App Shortcuts.
- Xcode 15.
- StoreKit for SwiftUI.
- Observation in SwiftUI.
- Advanced animations in SwiftUI.
- StoreKit 2 and StoreKit Testing in Xcode.
- UIKit.
- Design and build apps for watchOS 10.
- MapKit for SwiftUI.
- Sync to iCloud with CKSyncEngine.
- Web apps.
- Unleash the UIKit trait system.
- Design widgets for the Smart Stack on Apple Watch.
- Build programmatic UI with Xcode Previews.
- Explore advances in declarative device management.
- Beyond scroll views.
- Take SwiftUI to the next dimension.
- Push Notifications Console.
- Calendar and EventKit.
- App Store pricing.
- Rediscover Safari developer features.
- Bring widgets to life.
- Update apps for watchOS 10.
- CSS.
- AppKit.
- App Store server APIs.
- Create animated symbols.
- Simplify distribution in Xcode Cloud.
- Verify app dependencies with digital signatures.
- SwiftData.
- Inspectors in SwiftUI: Discover the details.
- Object Capture for iOS.
- App Store Connect.
- Demystify SwiftUI performance.
- Analyze hangs with Instruments.
Contacts
I have a clear focus on time-to-market and don't prioritize technical debt. And I took part in the Pre-Sale/RFX activity as a System Architect, assessment efforts for Mobile (iOS-Swift, Android-Kotlin) and Backend (NodeJS-.NET-PHP-Kafka-SQL-NoSQL). And I also formed the work of Pre-Sale as a CTO from Opportunity to Proposal via knowledge transfer to Successful Delivery.
π©οΈ #startups #management #cto #swift #typescript #database
π§ Email: sergey.leschev@gmail.com
π LinkedIn: https://linkedin.com/in/sergeyleschev
π Twitter: https://twitter.com/sergeyleschev
π Github: https://github.com/sergeyleschev
π Website: https://sergeyleschev.github.io
π DEV Community: https://dev.to/sergeyleschev
π Reddit: https://reddit.com/user/sergeyleschev
π Quora: https://quora.com/sergey-leschev
π Medium: https://medium.com/@sergeyleschev
π¨οΈ PDF: Download
ALT: SIARHEI LIASHCHOU